Tutorial: Random Control¶
1. No random control¶
[14]:
import matplotlib.pyplot as plt
import beacon_aug as BA
import numpy as np
from skimage import io
image = io.imread("../data/example.png")
aug_pipeline = BA.Compose([
BA.Rotate(p=1,limit=(-90,90), library= "albumentations")
])
augmented_image1 = aug_pipeline(image=image)['image']
augmented_image2 = aug_pipeline(image=image)['image']
# Display the result
plt.figure();plt.title("No random control: different results")
plt.imshow(np.hstack([ augmented_image1, augmented_image2]))
[14]:
<matplotlib.image.AxesImage at 0x7f8319cd40d0>
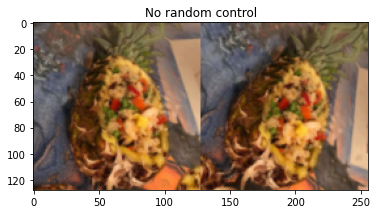
2. With hard random control:¶
add BA.properties.set_seed(100)
before calling the pipeline
[17]:
import matplotlib.pyplot as plt
import beacon_aug as BA
import numpy as np
from skimage import data
image = io.imread("../data/example.png")
aug_pipeline = BA.Compose([
BA.Rotate(p=1,limit=(-90,90), library= "albumentations")
])
# fix the random seed to fix the results
BA.properties.set_seed(100)
augmented_image1 = aug_pipeline(image=image)['image']
BA.properties.set_seed(100)
augmented_image2 = aug_pipeline(image=image)['image']
# Display the result
plt.figure();plt.title("With random control : same results")
plt.imshow(np.hstack([ augmented_image1, augmented_image2]))
[17]:
<matplotlib.image.AxesImage at 0x7f8319cc0b50>
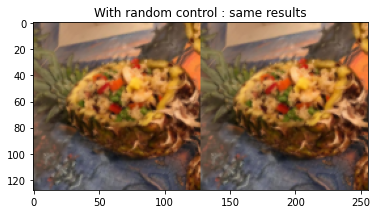
3. With soft random control:¶
[31]:
import matplotlib.pyplot as plt
import beacon_aug as BA
import numpy as np
from skimage import data
image = io.imread("../data/example.png")
# wide range of rotation limitations
aug_pipeline1 = BA.Compose([
BA.Rotate(p=1,limit=(-90,90), library= "albumentations")
])
# narrow range of rotation limitations
aug_pipeline2 = BA.Compose([
BA.Rotate(p=1,limit=(-30,30), library= "albumentations")
])
ls_1, ls_2 = [],[]
for i in range(5):
BA.properties.set_seed(i)
augmented_image1 = aug_pipeline1(image=image)['image']
ls_1.append(augmented_image1)
BA.properties.set_seed(i)
augmented_image2 = aug_pipeline2(image=image)['image']
ls_2.append(augmented_image2)
# Display the result
fig, axs = plt.subplots(2,1)
plt.suptitle("With soft random control ")
axs[0].imshow(np.hstack(ls_1))
axs[0].axis("off")
axs[1].imshow(np.hstack(ls_2))
axs[1].axis("off")
[31]:
(-0.5, 639.5, 127.5, -0.5)
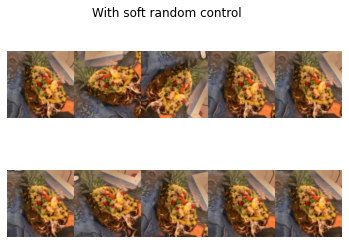
[ ]: